mirror of
https://github.com/jetzig-framework/jetzig.git
synced 2025-07-02 22:16:07 +00:00
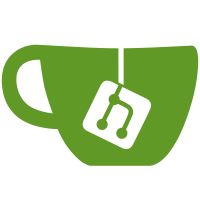
Use Karl Seguin's http.zig as HTTP server backend: https://github.com/karlseguin/http.zig Update loggers to use new `jetzig.loggers.LogQueue` to offload logging to a background thread. Numerous other optimizations to remove unneeded allocs. Performance jump on a simple request from approx. 2k requests/second to approx. 40k requests/second (Intel(R) Core(TM) i7-6700K CPU @ 4.00GHz). Color support for Windows Zmpl partial arg type coercion
45 lines
1.7 KiB
Zig
45 lines
1.7 KiB
Zig
const std = @import("std");
|
|
const jetzig = @import("../../jetzig.zig");
|
|
|
|
const Self = @This();
|
|
|
|
/// Initialize htmx middleware.
|
|
pub fn init(request: *jetzig.http.Request) !*Self {
|
|
const middleware = try request.allocator.create(Self);
|
|
return middleware;
|
|
}
|
|
|
|
/// Detects the `HX-Request` header and, if present, disables the default layout for the current
|
|
/// request. This allows a view to specify a layout that will render the full page when the
|
|
/// request doesn't come via htmx and, when the request does come from htmx, only return the
|
|
/// content rendered directly by the view function.
|
|
pub fn afterRequest(self: *Self, request: *jetzig.http.Request) !void {
|
|
_ = self;
|
|
if (request.headers.get("HX-Target")) |target| {
|
|
try request.server.logger.DEBUG(
|
|
"[middleware-htmx] htmx request detected, disabling layout. (#{s})",
|
|
.{target},
|
|
);
|
|
request.setLayout(null);
|
|
}
|
|
}
|
|
|
|
/// If a redirect was issued during request processing, reset any response data, set response
|
|
/// status to `200 OK` and replace the `Location` header with a `HX-Redirect` header.
|
|
pub fn beforeResponse(self: *Self, request: *jetzig.http.Request, response: *jetzig.http.Response) !void {
|
|
_ = self;
|
|
if (response.status_code != .moved_permanently and response.status_code != .found) return;
|
|
if (request.headers.get("HX-Request") == null) return;
|
|
|
|
if (response.headers.get("Location")) |location| {
|
|
response.status_code = .ok;
|
|
request.response_data.reset();
|
|
try response.headers.append("HX-Redirect", location);
|
|
}
|
|
}
|
|
|
|
/// Clean up the allocated htmx middleware.
|
|
pub fn deinit(self: *Self, request: *jetzig.http.Request) void {
|
|
request.allocator.destroy(self);
|
|
}
|