mirror of
https://github.com/jetzig-framework/jetzig.git
synced 2025-07-01 21:46:09 +00:00
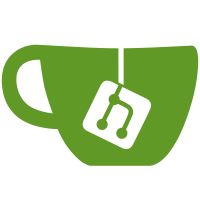
Eradication of `data` arg to requests. We no longer need to pass this value around as we have a) type inference, b) nested object insertion via `put` and `append`. Fix `joinPath` numeric type coercion Detect empty string params and treat them as blank with expectParams() Fix error logging/stack trace printing. Update Zmpl - includes debug tokens + error tracing to source template in debug builds.
34 lines
963 B
Zig
34 lines
963 B
Zig
const std = @import("std");
|
|
const colors = @import("jetzig").colors;
|
|
|
|
const icons = .{
|
|
.check = "✅",
|
|
.cross = "❌",
|
|
};
|
|
|
|
/// Print a success confirmation.
|
|
pub fn printSuccess() void {
|
|
std.debug.print(" " ++ icons.check ++ "\n", .{});
|
|
}
|
|
|
|
/// Print a failure confirmation.
|
|
pub fn printFailure() void {
|
|
std.debug.print(" " ++ icons.cross ++ "\n", .{});
|
|
}
|
|
|
|
const PrintContext = enum { success, failure };
|
|
/// Print some output in with a given context to stderr.
|
|
pub fn print(comptime context: PrintContext, comptime message: []const u8, args: anytype) !void {
|
|
const writer = std.io.getStdErr().writer();
|
|
switch (context) {
|
|
.success => try writer.print(
|
|
std.fmt.comptimePrint("{s} {s}\n", .{ icons.check, colors.green(message) }),
|
|
args,
|
|
),
|
|
.failure => try writer.print(
|
|
std.fmt.comptimePrint("{s} {s}\n", .{ icons.cross, colors.red(message) }),
|
|
args,
|
|
),
|
|
}
|
|
}
|